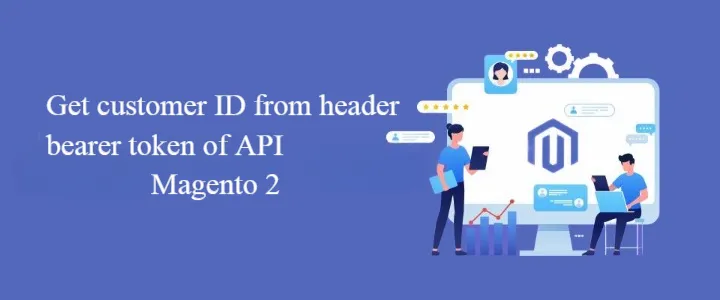
In this article, I will show you How to get customer id from header bearer token of API in Magento 2? In Magento 2, the customer ID can be retrieved from the Web API Header Authorization Token.
During the development, you need to retrieve the customer ID from the Bearer token included in the Header.
You can simply follow the provided code snippet to retrieve the customer ID from the current payload.You can just follow the given code snippet to fetch the customer ID from the current payload.
<?php
declare(strict_types=1);
namespace Vendor\Module\Webapi;
use Magento\Framework\Exception\AuthorizationException;
use Magento\Integration\Api\Exception\UserTokenException;
use Magento\Integration\Api\UserTokenReaderInterface;
use Magento\Integration\Api\UserTokenValidatorInterface;
use Magento\Framework\Webapi\Request;
class TokenCustomerId
{
public function __construct(
private readonly Request $request,
private readonly UserTokenReaderInterface $userTokenReader,
private readonly UserTokenValidatorInterface $userTokenValidator
) {
}
/**
* Get customer id based on the authorization token.
*
* @return int|null
* @throws AuthorizationException
*/
public function getCustomerIdByBearerToken(): ?int
{
$authorizationHeaderValue = $this->request->getHeader('Authorization');
if (!$authorizationHeaderValue) {
return null;
}
$headerPieces = explode(" ", $authorizationHeaderValue);
if (count($headerPieces) !== 2) {
return null;
}
$tokenType = strtolower($headerPieces[0]);
if ($tokenType !== 'bearer') {
return null;
}
$bearerToken = $headerPieces[1];
try {
$token = $this->userTokenReader->read($bearerToken);
} catch (UserTokenException $exception) {
throw new AuthorizationException(__($exception->getMessage()));
}
try {
$this->userTokenValidator->validate($token);
} catch (AuthorizationException $exception) {
return null;
}
return (int) $token->getUserContext()->getUserId();
}
}
With the above code, you can retrieve the current customer ID from the Authorization Header in the API request.
You may also like this: